Hi everyone,
I need assistance with creating a number card that dynamically updates the count of records based on the logged-in user. The goal is for each user to see the number of tickets assigned specifically to them.
For example:
- USER1 has 2 assigned tickets, so the number card should display a count of 2.
- USER2 has 3 assigned tickets, so the number card should display a count of 3.
Currently, I’m encountering an issue where the same count is displayed for all users, which is not the desired outcome. Could you please guide me on how to achieve this functionality?
Thank you in advance for your help!
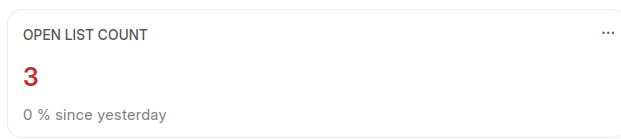
To create a number card in ERPNEXT that dynamically updates the count of records based on the logged-in user, you need to ensure that the count is filtered by the current user. Here’s a step-by-step guide on how to achieve this:
Step 1: Create a Custom Field for Assigned User
First, ensure that your “Ticket” doctype has a field to store the assigned user. This is usually a Link field to the “User” doctype.
- Go to Customize Form and select the “Ticket” doctype.
- Add a new field of type “Link”.
- Set the Options to “User” and label it as “Assigned To”.
Step 2: Create a Custom Script to Filter by Logged-in User
You will need to create a custom script that filters the count of tickets by the logged-in user. Here’s how you can achieve this:
- Go to Custom Script in ERPNEXT.
- Create a new Custom Script for the “Desk” (or the relevant doctype where your number card is displayed).
- Use the following script as a template:
frappe.ui.form.on('YourDoctype', {
refresh: function(frm) {
// Fetch count of tickets assigned to the logged-in user
frappe.call({
method: 'frappe.client.get_list',
args: {
doctype: 'Ticket',
filters: {
'assigned_to': frappe.session.user
},
fields: ['name']
},
callback: function(r) {
if (r.message) {
let count = r.message.length;
// Update the number card with the count
frm.dashboard.add_indicator(`Tickets Assigned: ${count}`, 'blue');
}
}
});
}
});
Step 3: Create a Dashboard Indicator
To display the count as a number card, you can create a custom dashboard indicator:
- Go to Dashboard and create a new dashboard if you don’t have one already.
- Create a new Dashboard Chart.
- Set the Chart Type to “Count”.
- Set the Document Type to “Ticket”.
- Set the Filter to:
- Field:
assigned_to
- Condition:
=
- Value:
{{ frappe.session.user }}
- Save the chart and add it to your dashboard.
Step 4: Customize the Dashboard for Dynamic Updates
For a more dynamic approach, ensure your dashboard updates in real-time or when the page is refreshed:
- Customize the dashboard to refresh based on certain triggers or intervals.
- Use the following code snippet to refresh the dashboard card:
frappe.dashboard.refresh = function() {
frappe.call({
method: 'frappe.desk.page.dashboard.dashboard.get_cards',
callback: function(r) {
r.message.cards.forEach(card => {
if (card.name === 'Tickets Assigned') {
card.value = r.message.cards.filter(card => card.fieldname === 'assigned_to' && card.value === frappe.session.user).length;
}
});
frappe.dashboard.render_cards(r.message.cards);
}
});
};